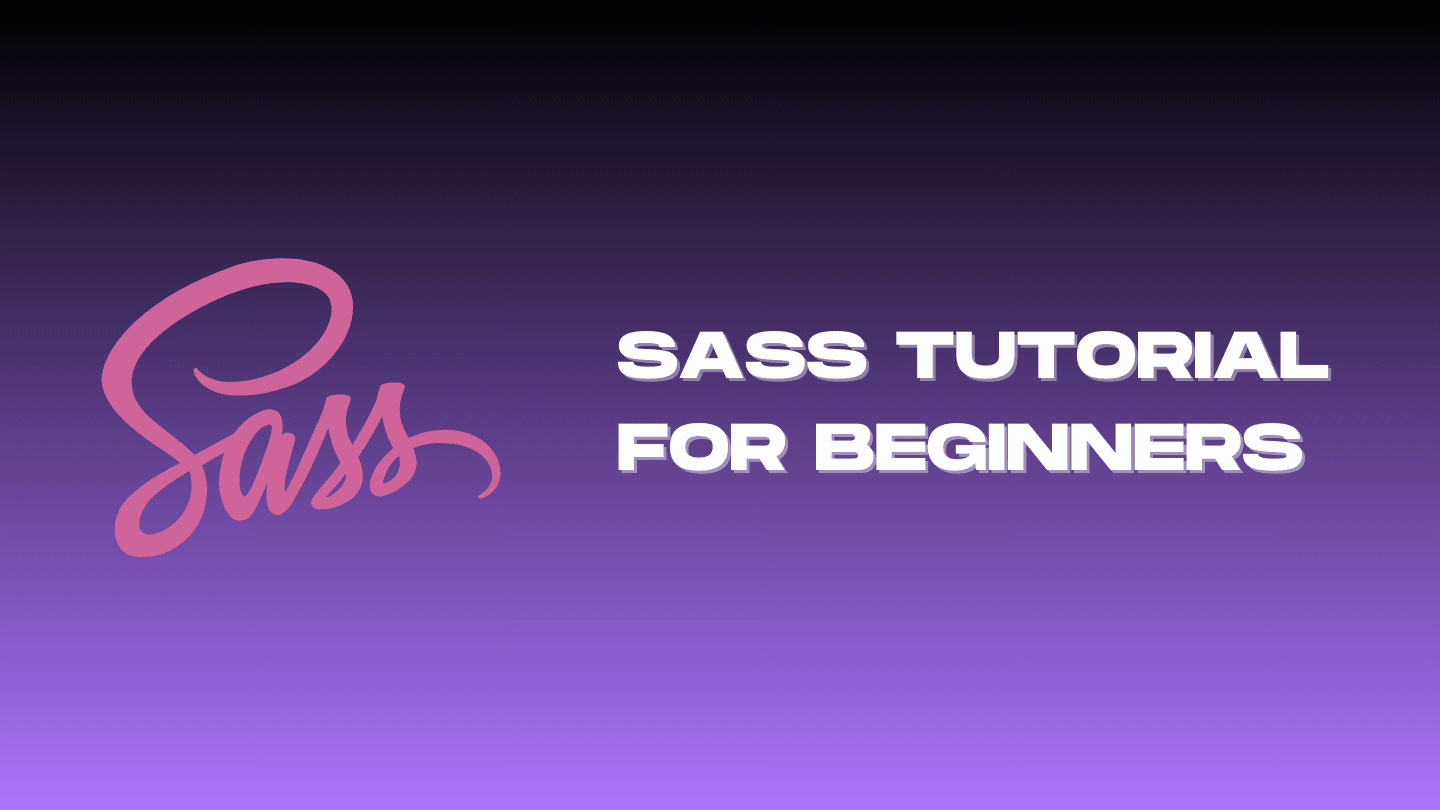
SASS Tutorial For Beginners
Dec 01 2024
SASS, or Syntactically Awesome Style Sheets, is a pre-processor scripting language that compiles into CSS. SASS extends the capabilities of CSS, offering powerful features that enable you to create styles in a more organized, modular, and maintainable way.
SASS vs. SCSS
SASS actually comes in two versions: the original SASS and the more popular SCSS. Both offer the same features, but the main difference lies in their syntax.
The SASS version is known as the indented syntax. It does not use curly braces {}
or semicolons ;
. Instead, it relies on indentation (similar to Python) to nest and structure styles.
$primary-color: #3498db
$font-stack: Arial, sans-serif
body
font-family: $font-stack
color: $primary-color
h1
font-size: 2rem
color: darken($primary-color, 10%)
SCSS, or Sassy CSS, on the other hand, uses the standard CSS syntax with curly braces {}
and semicolons ;
to define blocks of code.
$primary-color: #3498db;
$font-stack: Arial, sans-serif;
body {
font-family: $font-stack;
color: $primary-color;
h1 {
font-size: 2rem;
color: darken($primary-color, 10%);
}
}
Additionally, SASS files uses the .sass
extension, while SCSS files uses .scss
. This difference helps you know which one you're dealing with at a glance.
How To Compile SASS to CSS
Since browsers don’t understand SASS directly; it must be compiled into CSS. You can achieve this using a SASS command-line tool, a build tool, or an extension in your code editor.
Using the SASS Command Line
For example If you have Nodejs installed on your computer, globally install SASS using npm.
npm install -g sass
Then, in your project directory, you can easily compile your SASS files into CSS by running the following command in your terminal.
sass input.scss output.css
Here, input.scss
specifies the location of your SASS file, while output.css
indicates the desired location for the compiled CSS file.
Using the VS Code Extension
Furthermore, if you use Visual Studio Code as your editor, you can install the Live Sass Compiler extension to automatically compile your SASS files into CSS.
Once you install the extension, click the "Watch SASS" button at the bottom. This will automatically trigger the compilation process whenever you modify your SASS files. Sometimes the button may not appear; in this case, remember to try refreshing your editor.
After compiling is complete, you can see the generated CSS files in your project directory.
Features
Now, let's see features SASS offers.
Variables
One of the standout features of SASS is the use of variables. In SASS, variables are declared with a dollar sign ($) followed by the variable name, and then assigned a value.
You can define variables for colors, fonts, sizes, etc. so that you can easily change values in one place without editing your entire stylesheet.
$primary-color: #3498db;
$font-stack: 'Helvetica Neue', Arial, sans-serif;
body {
font-family: $font-stack;
background-color: $primary-color;
}
While CSS now supports variables, SASS variables are still useful for organizing and managing your styles.
Nesting
In SASS, you can nest CSS selectors in the way that mirrors the HTML structure. This approach lets you create hierarchically structured selectors for complex layouts, eliminating the need to repeatedly write parent selectors.
.nav {
background-color: #333;
ul {
list-style: none;
li {
display: inline-block;
a {
color: white;
text-decoration: none;
}
}
}
}
When nesting, the ampersand symbol, known as the "parent selector," makes it easy to reference the parent selector. It is useful for pseudo-classes, pseudo-elements, and more advanced selector combinations.
.button {
background-color: #3498db;
color: white;
padding: 10px 20px;
&.success {
background-color: #2ecc71;
}
&:hover {
background-color: darken(#3498db, 10%);
}
&--large {
padding: 40px;
}
}
.card {
padding: 20px;
background-color: #f5f5f5;
&--large {
padding: 40px;
}
&--primary {
background-color: #3498db;
}
}
SASS code above compiles into this:
.button {
background-color: #3498db;
color: white;
padding: 10px 20px;
}
.button.success {
background-color: #2ecc71;
}
.button:hover {
background-color: #2a80b9;
}
.button--large {
padding: 40px;
}
While CSS now also supports nesting, the way it handles the parent selector differs slightly. In native CSS nesting, the ampersand symbol must be used at the start to define a rule and you can not concatenate it with other selectors freely.
.button {
color: white;
&--large { /* This will not work in native CSS nesting */
padding: 20px;
}
&.success { /* This will not work either */
background-color: green;
}
}
Mixins
Mixins in SASS allow you to define reusable chunks of CSS that you can include throughout your stylesheet.
A mixin is created using the @mixin directive followed by the mixin's name and is included in styles with the @include directive, specifying the mixin's name.
@mixin rounded-corners {
border-radius: 10px;
}
.box {
@include rounded-corners;
}
In addition, A mixin can take parameters, making them even more flexible.
@mixin box-style($bg-color, $padding: 15px) {
background-color: $bg-color;
padding: $padding;
border-radius: 5px;
}
.card {
@include box-style(#3498db, 20px);
}
.alert {
@include box-style(#e74c3c);
}
Mixins are especially helpful for repetitive styles like vendor prefixes, button styles, or responsive breakpoints.
Functions
SASS allows you to define custom functions that return values. A function is defined using the @function directive, followed by its name and parameters. The @return directive specifies the value to be returned.
You can use functions in SASS to perform calculations, manipulate colors, or handle other operations that help keep your stylesheets flexible and clean.
@function grid-width($columns, $gutter: 10px) {
@return ($columns * 100px) + (($columns - 1) * $gutter);
}
.grid-item {
width: grid-width(3); // Outputs 320px
}
Moreover, Sass provides built-in functions that make it easier to manipulate colors, numbers, strings, and more. You can use them by simply calling their names in your styles.
$primary-color: #3498db;
.button {
background-color: lighten($primary-color, 10%);
color: darken($primary-color, 20%);
}
If-Else
In SASS, you can use conditional statements like @if, @else if, and @else to control the flow of your styles based on conditions. This is helpful for applying different styles depending on specific criteria, such as breakpoints, themes, or other design choices.
@mixin button-style($type) {
padding: 10px 20px;
border-radius: 5px;
@if $type == primary {
background-color: #3498db;
color: #fff;
} @else if $type == secondary {
background-color: #2ecc71;
color: #fff;
} @else {
background-color: #ccc;
color: #333;
}
}
.button-primary {
@include button-style(primary);
}
.button-secondary {
@include button-style(secondary);
}
Loops
SASS provides powerful looping constructs that allow you to repeat styles or generate multiple classes dynamically. The most commonly used loops in SASS are @for, @each, and @while. These constructs help reduce redundancy and create complex stylesheets more efficiently.
@for Loop
The @for loop iterates over a range of numbers, allowing you to create styles based on a sequence.
$columns: 4;
@for $i from 1 through $columns {
.column-#{$i} {
width: 100% / $columns;
}
}
.column-1 {
width: 25%;
}
.column-2 {
width: 25%;
}
.column-3 {
width: 25%;
}
.column-4 {
width: 25%;
}
@each Loop
The @each loop iterates over a list or map, which is great for applying styles to multiple items.
$colors: red, green, blue;
@each $color in $colors {
.bg-#{$color} {
background-color: $color;
}
}
.bg-red {
background-color: red;
}
.bg-green {
background-color: green;
}
.bg-blue {
background-color: blue;
}
@while Loop
The @while loop continues until a specified condition is false. It is less commonly used but can be handy for certain situations.
$count: 1;
@while $count <= 5 {
.item-#{$count} {
width: $count * 20px;
}
$count: $count + 1;
}
.item-1 {
width: 20px;
}
.item-2 {
width: 40px;
}
.item-3 {
width: 60px;
}
.item-4 {
width: 80px;
}
.item-5 {
width: 100px;
}
Partials
SASS partials are a way to organize your stylesheets into smaller, manageable pieces. By breaking down your styles into partial files, you can make your code more modular and easier to maintain. Partials are typically used for different components or sections of a website, like headers, footers, buttons, and so on.
// _variables.scss
$primary-color: #3498db;
$font-stack: Arial, sans-serif;
// main.scss
@import 'variables';
@import 'mixins';
@import 'buttons';
// You can also use the styles here directly
body {
font-family: $font-stack;
}
Conclusion
SASS provides a powerful set of tools to enhance your CSS workflow. By incorporating variables, nesting, mixins, and more, you can write cleaner, more maintainable stylesheets
Thank you for reading.