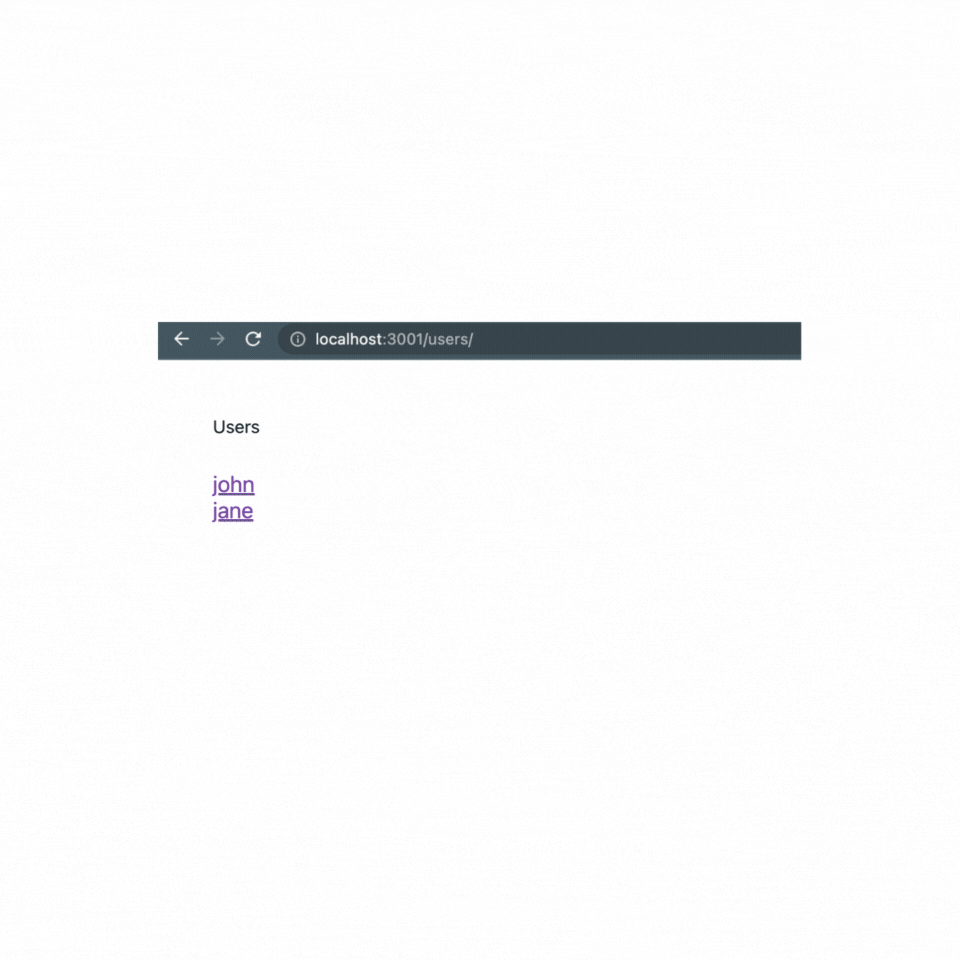
Nested Routes With React Router V6
By onjsdev
Nov 26th, 2023
Nested routes in React allow fragments or tabs to be injected into the page and changed URLs at the same time without completely redirecting the user to another page.
In React, static and dynamic nested routes can be both implemented. For example, when dealing with a large number of users, it's impractical to create separate static routes for each one. Instead, you can establish a single route for dynamically changing URLs and injecting data in a fragment or tab into the page.
Now, let's see the first step on how to create nested routes in react using react router v6.
Install React Router v6
Before you start, make sure you have installed react router packages using the following command.
npm i react-router react-router-dom
How To Set Nested Routes
We will set three different types of routes and their components. One of them is parent users
, other one is nested dynamic child route :user
, the last one is static create
route.
import "./App.css";
import { BrowserRouter, Routes, Route } from "react-router-dom";
import Users from "./components/Users";
import User from "./components/User";
import Create from "./components/Create";
function App() {
return (
<BrowserRouter>
<Routes>
<Route path="/users" element={<Users />}>
{/* Dynamic Nested Route */}
<Route path=":user" element={<User />} />
{/* Static Nested Route */}
<Route path="create" element={<Create />} />
</Route>
</Routes>
</BrowserRouter>
);
}
export default App;
How To Implement Parent Route
In our parent users component, we will render all users. When one of them is clicked, the child nested :user
component will be injected to the page with the help of Outlet
component from the react-router
package.
For example, when an user visit the page /users/john
, the User
will be rendered with the corresponding data of the user John.
import React from "react";
import { Link, Outlet } from "react-router-dom";
export default function Users() {
const users = [
{ id: "1", name: "john" },
{ id: "2", name: "jane" },
];
return (
<div className="users">
Users
{/* List all users */}
<div className="list">
{users.map((user) => (
<Link className="user" to={user.name} key={user.id}>
{user.name}
</Link>
))}
</div>
{/* When clicked a user, inject its data into here */}
<Outlet></Outlet>
</div>
);
}
How To Create Nested Dynamic Component
As mentioned earlier, in our dynamic nested routes will render a dynamic User
component. Using the useParams
hook, this component will get the the name of the user from the URL and simply display that name on the screen.
Of course, the data for this user can be fetched from server and display it, however we simply render the name for simplicity.
import React from "react";
import { useParams } from "react-router-dom";
function User() {
const { user } = useParams();
return <div>{user} is selected</div>;
}
export default User;
How To Create Nested Static Route Component
We also have a static route called create
to create a new user. For the sake of simplicity we will only render a simple HTML form instead of submitting the info of the user to a server.
import React from "react";
export default function Create() {
return (
<div>
<h1>Create A User</h1>
<input placeholder="A User Name" />
</div>
);
}
When a user will visit the route /users/create
, this component will be rendered.
Conclusion
That's all. In this guide, we talked about dynamic and static nested routes with react router v6, which enables creating of tab-based pages. After setting the routes, you can test the URLs by attempting navigate a nested route.
Thank you for reading.